JavaScript字符串用于存储和处理文本。它可以在引号中包含零个或多个字符。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings</h2>
<p id= "GFG" ></p>
<!-- Script to store string in variable -->
<script>
// String written inside quotes
var x = "Welcome to lsbin!" ;
document.getElementById( "GFG" ).innerHTML = x;
</script>
</body>
</html>
输出如下:
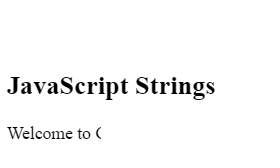
实现字符串的方法:下面主要列出两种实现字符串的方法。
范例1:
使用单引号或双引号来编写字符串。
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings</h2>
<p id= "GFG" ></p>
<!-- Script to initialize string -->
<script>
var x = "lsbin" ;
var y = 'A computer science portal' ;
document.getElementById( "GFG" ).innerHTML =
x + "<br>" + y;
</script>
</body>
</html>
输出如下:
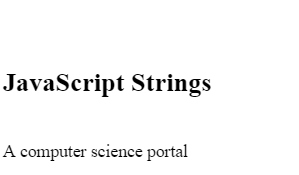
范例2:
只要引号与字符串周围的引号不匹配, 就可以在字符串内使用引号。
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings</h2>
<p id= "GFG" ></p>
<script>
var x = "'lsbin'" ;
var y = "A 'computer' 'science' portal" ;
document.getElementById( "GFG" ).innerHTML =
x + "<br>" + y;
</script>
</body>
</html>
输出如下:
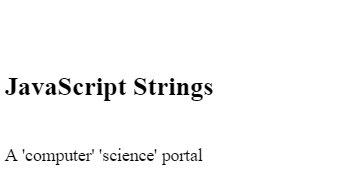
特殊的角色:如上所述, 特殊字符不能在字符串中使用相同类型的引号, 但是有一个解决方案。它使用反斜杠转义字符。反斜杠" \"转义字符将特殊字符转换为普通字符串字符。序列(\")用于在字符串中插入双引号。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings for special character</h2>
<p id= "GFG" ></p>
<!-- Script to use special character -->
<script>
var x = "\"lsbin\" A \'computer science\' portal" ;
document.getElementById( "GFG" ).innerHTML = x;
</script>
</body>
</html>
输出如下:
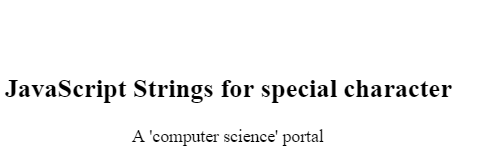
例子:
字符串可以用单引号引起来。
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings for special character</h2>
<p id= "GFG" ></p>
<!-- Script to use special character -->
<script>
var x = '\"lsbin\" A \'computer science\' portal' ;
document.getElementById("GFG").innerHTML = x;
</script>
</body>
</html>
输出如下:
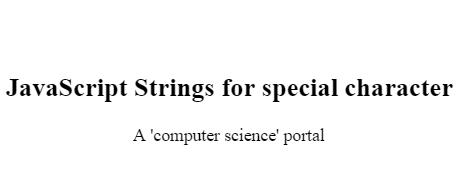
字串长度:字符串的长度可以使用长度属性。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings length</h2>
<p id= "GFG" ></p>
<!-- Script to return the length of string -->
<script>
var len = "lsbin" ;
// Returns the length of string
document.getElementById( "GFG" ).innerHTML
= len.length;
</script>
</body>
</html>
输出如下:
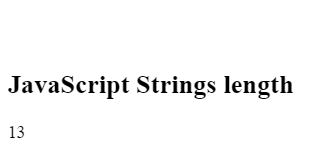
断字符串:有时为了便于理解, 我们需要将字符串分开, 符号\可以使用, 但不是首选。首选方法是使用+两个字符串之间的符号。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings break lines</h2>
<p id= "GFG" ></p>
<!-- Script to break the line -->
<script>
document.getElementById( "GFG" ).innerHTML = "Welcome"
+ " to lsbin!" ;
</script>
</body>
</html>
输出如下:
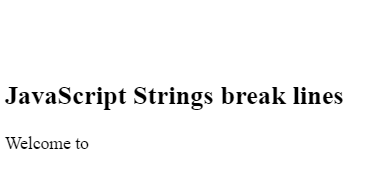
字符串作为对象:通过使用关键字" new", 可以将字符串用作对象。
例子:
<!DOCTYPE html>
<html>
<head>
<title>
JavaScript Strings
</title>
</head>
<body>
<h1>lsbin</h1>
<h2>JavaScript Strings as object</h2>
<p id= "GFG" ></p>
<!-- Script to use string as object -->
<script>
// Declare a string
var x = "Great Geek" ;
// Declare an object
var y = new String( "Great Geek" );
document.getElementById( "GFG" ).innerHTML =
typeof x + "<br>" + typeof y;
</script>
</body>
</html>
输出如下:
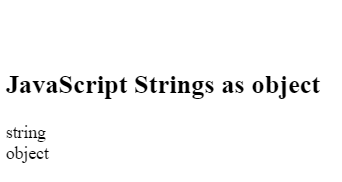