Python使用了不同的GUI应用程序, 这些应用程序在与用户使用的应用程序进行交互时对用户很有帮助。 python基本上使用三个GUITkinter, wxPython和PyQt。所有这些都可以在Windows, Linux和mac-OS上运行。但是, 这些GUI应用程序具有许多小部件, 即有助于用户与应用程序交互的控件。其中的一些小部件是按钮, 列表框, 滚动条, 树状视图等。
注意:有关更多信息, 请参阅到Python GUI – tkinter
Treeview小部件
该小部件有助于可视化并允许在项目的层次结构上进行导航。它可以显示层次结构中每个项目的多个功能。它可以像在Windows资源管理器中一样构建类似于用户界面的树形视图。因此, 在这里我们将使用Tkinter以便在Python GUI应用程序中构造层次结构树视图。
让我们来看一个构造一个 Python GUI应用程序中的分层树视图
例子:
# Python program to illustrate the usage
# of hierarchical treeview in python GUI
# application using tkinter
# Importing tkinter
from tkinter import *
# Importing ttk from tkinter
from tkinter import ttk
# Creating app window
app = Tk()
# Defining title of the app
app.title( "GUI Application of Python" )
# Defining label of the app and calling a geometry
# management method i.e, pack in order to organize
# widgets in form of blocks before locating them
# in the parent widget
ttk.Label(app, text = "Treeview(hierarchical)" ).pack()
# Creating treeview window
treeview = ttk.Treeview(app)
# Calling pack method on the treeview
treeview.pack()
# Inserting items to the treeview
# Inserting parent
treeview.insert(' ', ' 0 ', ' item1', text = 'lsbin' )
# Inserting child
treeview.insert(' ', ' 1 ', ' item2', text = 'Computer Science' )
treeview.insert(' ', ' 2 ', ' item3', text = 'GATE papers' )
treeview.insert(' ', ' end ', ' item4', text = 'Programming Languages' )
# Inserting more than one attribute of an item
treeview.insert( 'item2' , 'end' , 'Algorithm' , text = 'Algorithm' )
treeview.insert( 'item2' , 'end' , 'Data structure' , text = 'Data structure' )
treeview.insert( 'item3' , 'end' , '2018 paper' , text = '2018 paper' )
treeview.insert( 'item3' , 'end' , '2019 paper' , text = '2019 paper' )
treeview.insert( 'item4' , 'end' , 'Python' , text = 'Python' )
treeview.insert( 'item4' , 'end' , 'Java' , text = 'Java' )
# Placing each child items in parent widget
treeview.move( 'item2' , 'item1' , 'end' )
treeview.move( 'item3' , 'item1' , 'end' )
treeview.move( 'item4' , 'item1' , 'end' )
# Calling main()
app.mainloop()
输出如下:
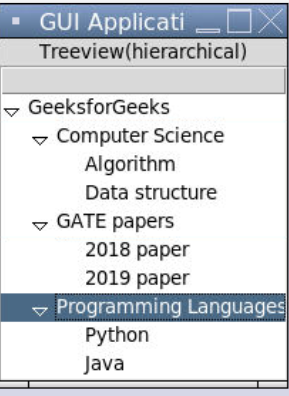
在上面的输出中, 创建了一个层次树视图。哪里, 极客是父母计算机科学, GATE论文和编程语言作为它的孩子。所有的孩子都有各自的属性。最后, 移动()为了将所有子级连接到父树, 在这里调用方法。
首先, 你的面试准备可通过以下方式增强你的数据结构概念:Python DS课程。