static是Java中的不可访问修饰符, 适用于以下情况:
- 块block(静态块)
- 变量
- 方法
- 嵌套类
要创建静态成员(块, 变量, 方法, 嵌套类), 请在其声明之前添加关键字static。将成员声明为静态成员后, 可以在创建其类的任何对象之前对其进行访问, 并且无需引用任何对象。例如, 在下面的Java程序中, 我们正在访问静态方法m1()而不创建任何对象测试类。
// Java program to demonstrate that a static member
// can be accessed before instantiating a class
class Test
{
// static method
static void m1()
{
System.out.println( "from m1" );
}
public static void main(String[] args)
{
// calling m1 without creating
// any object of class Test
m1();
}
}
输出如下:
from m1
静态块
如果你需要进行计算以初始化你的静态变量, 你可以声明一个静态块, 该类在首次加载该类时仅执行一次。考虑以下演示静态块用法的java程序。
// Java program to demonstrate use of static blocks
class Test
{
// static variable
static int a = 10 ;
static int b;
// static block
static {
System.out.println( "Static block initialized." );
b = a * 4 ;
}
public static void main(String[] args)
{
System.out.println( "from main" );
System.out.println( "Value of a : " +a);
System.out.println( "Value of b : " +b);
}
}
输出如下:
Static block initialized.
from main
Value of a : 10
Value of b : 40
有关静态块的详细文章, 请参见静态块
当变量声明为静态变量时, 将创建该变量的单个副本并在类级别的所有对象之间共享。本质上, 静态变量是全局变量。该类的所有实例共享相同的静态变量。
静态变量的重点:
- 我们只能在类级别创建静态变量。看到这里
- 静态块和静态变量按它们在程序中的顺序执行。
下面的Java程序演示了静态块和静态变量按它们在程序中的顺序执行。
// java program to demonstrate execution
// of static blocks and variables
class Test
{
// static variable
static int a = m1();
// static block
static {
System.out.println( "Inside static block" );
}
// static method
static int m1() {
System.out.println( "from m1" );
return 20 ;
}
// static method(main !!)
public static void main(String[] args)
{
System.out.println( "Value of a : " +a);
System.out.println( "from main" );
}
}
输出如下:
from m1
Inside static block
Value of a : 20
from main
静态方法
当方法声明为static关键字, 称为静态方法。静态方法最常见的示例是main()如上所述, 在创建其类的任何对象之前都可以访问任何静态成员, 并且无需引用任何对象。声明为static的方法有几个限制:
- 他们只能直接调用其他静态方法。
- 他们只能直接访问静态数据。
- 他们无法参考这个or超以任何方式。
以下是演示静态方法限制的Java程序。
// java program to demonstrate restriction on static methods
class Test
{
// static variable
static int a = 10 ;
// instance variable
int b = 20 ;
// static method
static void m1()
{
a = 20 ;
System.out.println( "from m1" );
// Cannot make a static reference to the non-static field b
b = 10 ; // compilation error
// Cannot make a static reference to the
// non-static method m2() from the type Test
m2(); // compilation error
// Cannot use super in a static context
System.out.println( super .a); // compiler error
}
// instance method
void m2()
{
System.out.println( "from m2" );
}
public static void main(String[] args)
{
// main method
}
}
何时使用静态变量和方法?
将静态变量用于所有对象共有的属性。例如, 在"学生"类中, 所有学生都使用相同的大学名称。使用静态方法来更改静态变量。
考虑以下java程序, 该程序说明了static带有变量和方法的关键字。
// A java program to demonstrate use of
// static keyword with methods and variables
// Student class
class Student
{
String name;
int rollNo;
// static variable
static String cllgName;
// static counter to set unique roll no
static int counter = 0 ;
public Student(String name)
{
this .name = name;
this .rollNo = setRollNo();
}
// getting unique rollNo
// through static variable(counter)
static int setRollNo()
{
counter++;
return counter;
}
// static method
static void setCllg(String name){
cllgName = name ;
}
// instance method
void getStudentInfo(){
System.out.println( "name : " + this .name);
System.out.println( "rollNo : " + this .rollNo);
// accessing static variable
System.out.println( "cllgName : " + cllgName);
}
}
//Driver class
public class StaticDemo
{
public static void main(String[] args)
{
// calling static method
// without instantiating Student class
Student.setCllg( "XYZ" );
Student s1 = new Student( "Alice" );
Student s2 = new Student( "Bob" );
s1.getStudentInfo();
s2.getStudentInfo();
}
}
输出如下:
name : Alice
rollNo : 1
cllgName : XYZ
name : Bob
rollNo : 2
cllgName : XYZ
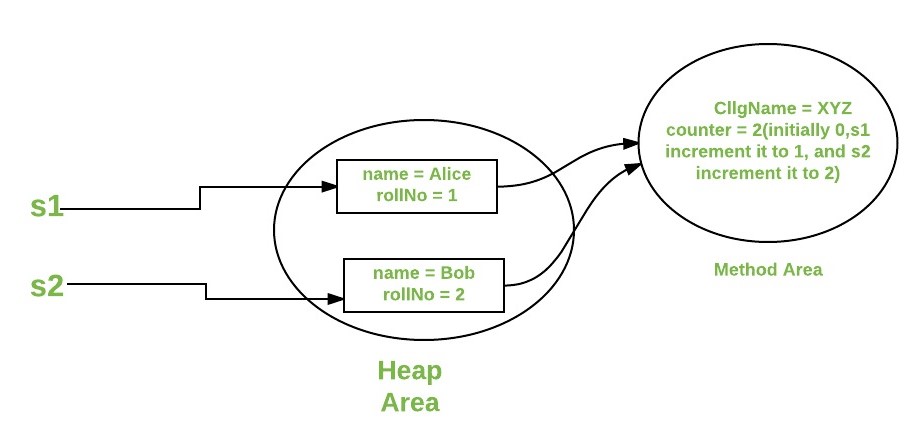
静态嵌套类:
我们不能使用静态修饰符声明顶级类, 但是可以声明嵌套类作为静态。这种类型的类称为嵌套静态类。有关静态嵌套类, 请参见Java中的静态嵌套类。
如果发现任何不正确的地方, 或者想分享有关上述主题的更多信息, 请写评论。