Javascript具有事件, 以提供网页的动态界面。这些事件与文档对象模型(DOM)中的元素挂钩。
这些事件默认情况下使用冒泡传播, 即在DOM中从子级到父级向上传播。我们可以将事件绑定为内联或外部脚本。
有一些javascript事件:
1)onclick事件:这是鼠标事件, 如果用户单击绑定到该元素的元素, 则将引发任何定义的逻辑。
代码1:
<!doctype html>
< html >
< head >
< script >
function hiThere() {
alert('Hi there!');
}
</ script >
</ head >
< body >
< button type = "button" onclick = "hiThere()" >Click me event</ button >
</ body >
</ html >
输出如下:
在点击"点击我的活动"键之前,
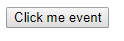
点击"点击我的活动"键后,
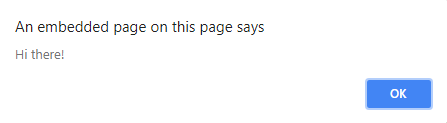
2)onkeyup事件:此事件是键盘事件, 在按下后释放键时会执行指令。
代码2:
<!doctype html>
< html >
< head >
< script >
var a = 0;
var b = 0;
var c = 0;
function changeBackground() {
var x = document.getElementById('bg');
bg.style.backgroundColor = 'rgb('+a+', '+b+', '+c+')';
a += 1;
b += a + 1;
c += b + 1;
if (a > 255) a = a - b;
if (b > 255) b = a;
if (c > 255) c = b;
}
</ script >
</ head >
< body >
< input id = "bg" onkeyup = "changeBackground()"
placeholder = "write something" style = "color:#fff" >
</ body >
</ html >
输出如下:
在写" gfg"之前-

写完" gfg"后
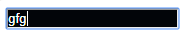
3)onmouseover事件:此事件对应于将鼠标指针悬停在绑定到其的元素及其子元素上。
代码3:
<!doctype html>
< html >
< head >
< script >
function hov() {
var e = document.getElementById('hover');
e.style.display = 'none';
}
</ script >
</ head >
< body >
< div id = "hover" onmouseover = "hov()"
style = "background-color:green;height:200px;width:200px;" >
</ div >
</ body >
</ html >
输出如下:
在将鼠标移到绿色方块之前,
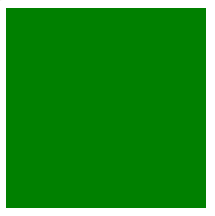
鼠标接管后, 绿色方块消失。
4)onmouseout事件:每当鼠标光标离开处理mouseout事件的元素时, 就会执行与其关联的功能。
代码4:
<!doctype html>
< html >
< head >
< script >
function out() {
var e = document.getElementById('hover');
e.style.display = 'none';
}
</ script >
</ head >
< body >
< div id = "hover" onmouseout = "out()"
style = "background-color:green;height:200px;width:200px;" >
</ div >
</ body >
</ html >
输出如下:
在将鼠标移到绿色方块上之前,
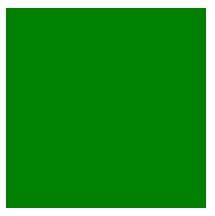
将鼠标移到上方并在一段时间后将其删除后, 绿色方块将消失。
5)onchange事件:此事件检测此事件的任何元素列表的值更改。
代码5:
<!doctype html>
< html >
< head ></ head >
< body >
< input onchange = "alert(this.value)" type = "number" >
</ body >
</ html >
输出如下:
在按下任何键之前-

按下2键后-
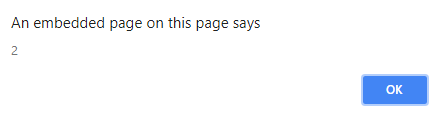
6)onload事件:元素完全加载后, 将引发此事件。
代码6:
<!doctype html>
< html >
< head ></ head >
< body >
< img onload = "alert('Image completely loaded')"
alt = "GFG-Logo"
src = "https://media.lsbin.org/wp-content/cdn-uploads/lsbinLogoHeader.png" >
</ body >
</ html >
输出如下:
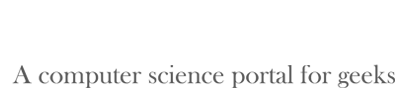
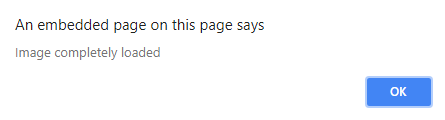
7)onfocus事件:列出此事件的元素在获得焦点时就会执行指令。
代码7:
<!doctype html>
<!doctype html>
< html >
< head >
< script >
function focused() {
var e = document.getElementById('inp');
if (confirm('Got it?')) {
e.blur();
}
}
</ script >
</ head >
< body >
< p >Take the focus into the input box below:</ p >
< input id = "inp" onfocus = "focused()" >
</ body >
</ html >
输出如下:
在框内单击鼠标之前,
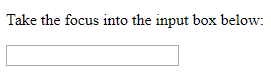
在框内单击鼠标后,
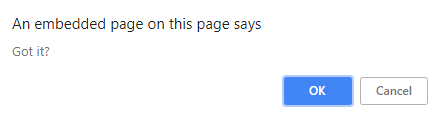
8)onblur事件:当元素失去焦点时会引发此事件。
代码8:
<!doctype html>
< html >
< head ></ head >
< body >
< p >Write something in the input box and then click elsewhere
in the document body.</ p >
< input onblur = "alert(this.value)" >
</ body >
</ html >
输出如下:
在框内输入" gfg"之前,

在框内输入" gfg"并按Enter键后,
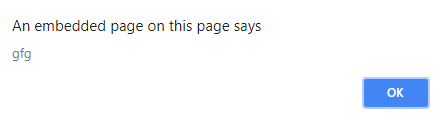
PS: onmouseup事件监听鼠标左键和中键点击,但onmousedown事件监听鼠标左键、中键和右键点击,而onclick只处理鼠标左键点击。。