本文概述
到目前为止, 使用Java程序的操作是在没有存储在任何地方的提示/终端上完成的。但是在软件行业, 大多数程序是编写来存储从程序中获取的信息的。一种这样的方法是将获取的信息存储在文件.
Java中的文件处理是什么?
一种文件是用于存储各种信息的容器。通过创建具有唯一名称的文件, 数据永久存储在辅助存储器中。文件可以包含文本, 图像或任何其他文档。
可以对文件执行的不同操作是:
- 创建一个新文件
- 打开现有文件
- 从文件读取
- 写入文件
- 移动到文件中的特定位置
- 关闭档案
Java中可用于文件处理的不同类:
- 输入流
- 输出流
- FilterOutputStream
- FileOutputStream
- ByteArrayOutputStream
- ByteArrayInputStream
- FileInputStream
- FilterInputStream
- StringBufferInputStream
- SequenceInputStream
- 缓冲输出流
- StringBufferInputStream
- 数据输出流
- 打印流
- BufferedInputStream
- 数据输入流
- PushbackInputStream
在本文中, 我们将学习如何Java字节流用于使用类执行8位(1字节)的输入和输出随机存取文件组成方法writeBytes()和readBytes()以字节形式写入和读取数据。
用于执行文件操作的各种方法:
- writeBytes(String s):将字符串作为字节序列写入文件。
- readLine():从此文件读取下一行文本。
- getFilePointer():返回此文件中的当前偏移量。
- 长度():返回此文件的长度, 返回类型为long。
- 关():关闭此随机访问文件流, 并释放与该流关联的所有系统资源。
- setLength(long newLength):设置此文件的长度。
- 搜寻(长位置):设置文件指针偏移量, 从该文件的开头开始测量, 在该位置下一次读取或写入。
Java中的文件打开模式:
值 | 含义 |
---|---|
" r" | 打开仅供阅读。调用结果对象的任何write方法将导致抛出IOException。 |
" rw" | 开放供阅读和写作。如果该文件尚不存在, 则将尝试创建它。 |
" rws" | 与" rw"一样, 它可以读取和写入, 并且还要求对文件内容或元数据的每次更新都必须同步写入底层存储设备。 |
" rwd" | 与" rw"一样, 可以进行读写操作, 并且还要求将文件内容的每次更新都同步写入基础存储设备。 |
使用RandomAccessFile打开文件的语法:
File file = new File( filename )
RandomAccessFile raf = new RandomAccessFile(file, mode)
使用Java中的文件处理进行CRUD操作
例子:考虑到你要在文件中保留朋友的联系电话的记录。要区分朋友的姓名和联系电话, 你需要使用分隔符。为此, 你需要选择一个分隔符, 例如'!'或'$'或某些没有出现在朋友名字中的特殊符号。然后, 我们将形成一个由名称, 特殊符号和数字组成的字符串, 以插入文件中。
文件friendsContact.txt中联系人的语法:
Name!Number
如何用Java创建文件?
// Java program to create a file "friendsContact.txt"
// and add a new contact in the file
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class AddFriend {
public static void main(String data[])
{
try {
// Get the name of the contact to be updated
// from the Command line argument
String newName = data[ 0 ];
// Get the number to be updated
// from the Command line argument
long newNumber = Long.parseLong(data[ 1 ]);
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Checking whether the name
// of contact already exists.
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number
= Long
.parseLong(
nameNumberString
.substring(index + 1 ));
// if condition to find existence of record.
if (name == newName || number == newNumber) {
found = true ;
break ;
}
}
if (found == false ) {
// Enter the if block when a record
// is not already present in the file.
nameNumberString
= newName
+ "!"
+ String.valueOf(newNumber);
// writeBytes function to write a string
// as a sequence of bytes.
raf.writeBytes(nameNumberString);
// To insert the next record in new line.
raf.writeBytes(System.lineSeparator());
// Print the message
System.out.println( " Friend added. " );
// Closing the resources.
raf.close();
}
// The contact to be updated
// could not be found
else {
// Closing the resources.
raf.close();
// Print the message
System.out.println( " Input name"
+ " does not exists. " );
}
}
catch (IOException ioe) {
System.out.println(ioe);
}
catch (NumberFormatException nef) {
System.out.println(nef);
}
}
}
输出如下:
在新创建的文件中编译并添加联系人:
javac AddFriend.java
java AddFriend abc 1111111111
Friend added
java AddFriend pqr 1111111111
Input name or number already exist
文件:
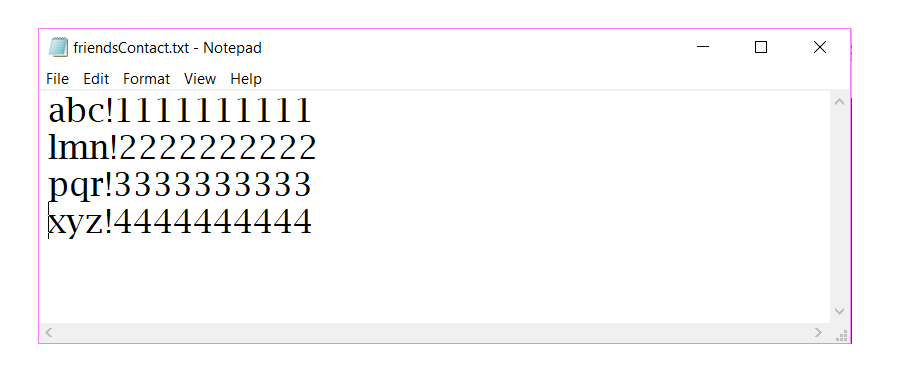
如何用Java读取文件?
// Java program to read from file "friendsContact.txt"
// and display the contacts
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class DisplayFriends {
public static void main(String data[])
{
try {
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Traversing the file
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number
= Long
.parseLong(
nameNumberString
.substring(index + 1 ));
// Print the contact data
System.out.println( "Friend Name: "
+ name + "\n"
+ "Contact Number: "
+ number + "\n" );
}
catch (IOException ioe)
{
System.out.println(ioe);
}
catch (NumberFormatException nef)
{
System.out.println(nef);
}
}
}
输出如下:
编译并从文件中读取联系人:
javac DisplayFriends.java
java DisplayFriends
Friend Name: abc
Contact Number: 1234567890
Friend Name: lmn
Contact Number: 3333333333
Friend Name: xyz
Contact Number: 4444444444
文件:
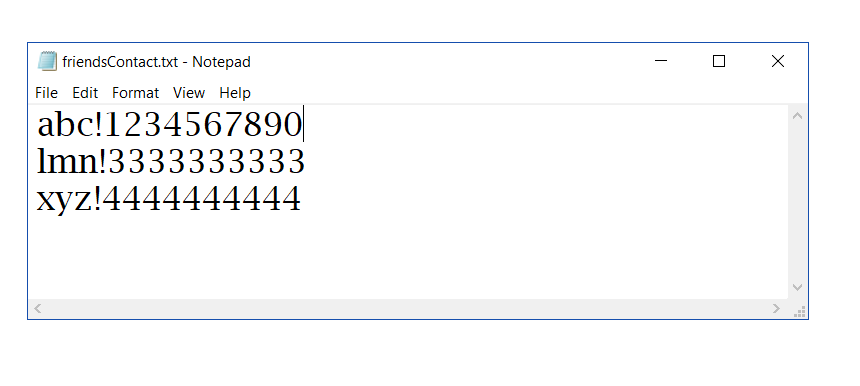
如何用Java更新文件?
// Java program to update in the file "friendsContact.txt"
// and change the number of an old contact
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class UpdateFriend {
public static void main(String data[])
{
try {
// Get the name of the contact to be updated
// from the Command line argument
String newName = data[ 0 ];
// Get the number to be updated
// from the Command line argument
long newNumber = Long.parseLong(data[ 1 ]);
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Checking whether the name
// of contact already exists.
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number = Long
.parseLong(nameNumberString
.substring(index + 1 ));
// if condition to find existence of record.
if (name == newName || number == newNumber) {
found = true ;
break ;
}
}
// Update the contact if record exists.
if (found == true ) {
// Creating a temporary file
// with file pointer as tmpFile.
File tmpFile = new File( "temp.txt" );
// Opening this temporary file
// in ReadWrite Mode
RandomAccessFile tmpraf
= new RandomAccessFile(tmpFile, "rw" );
// Set file pointer to start
raf.seek( 0 );
// Traversing the friendsContact.txt file
while (raf.getFilePointer() < raf.length()) {
// Reading the contact from the file
nameNumberString = raf.readLine();
index = nameNumberString.indexOf( '!' );
name = nameNumberString.substring( 0 , index);
// Check if the fetched contact
// is the one to be updated
if (name.equals(inputName)) {
// Update the number of this contact
nameNumberString
= name + "!"
+ String.valueOf(newNumber);
}
// Add this contact in the temporary file
tmpraf.writeBytes(nameNumberString);
// Add the line separator in the temporary file
tmpraf.writeBytes(System.lineSeparator());
}
// The contact has been updated now
// So copy the updated content from
// the temporary file to original file.
// Set both files pointers to start
raf.seek( 0 );
tmpraf.seek( 0 );
// Copy the contents from
// the temporary file to original file.
while (tmpraf.getFilePointer() < tmpraf.length()) {
raf.writeBytes(tmpraf.readLine());
raf.writeBytes(System.lineSeparator());
}
// Set the length of the original file
// to that of temporary.
raf.setLength(tmpraf.length());
// Closing the resources.
tmpraf.close();
raf.close();
// Deleting the temporary file
tmpFile.delete();
System.out.println( " Friend updated. " );
}
// The contact to be updated
// could not be found
else {
// Closing the resources.
raf.close();
// Print the message
System.out.println( " Input name"
+ " does not exists. " );
}
}
catch (IOException ioe) {
System.out.println(ioe);
}
catch (NumberFormatException nef) {
System.out.println(nef);
}
}
}
输出如下:
编译和更新文件中的联系人:
javac UpdateFriend.java
java UpdateFriend abc 1234567890
Friend updated.
java UpdateFriend tqr
Input name does not exists.
文件:
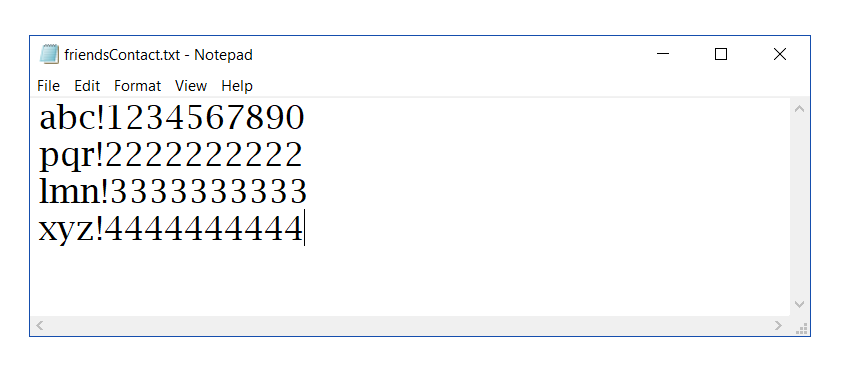
如何用Java删除文件?
// Java program to delete a contact
// from the file "friendsContact.txt"
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class DeleteFriend {
public static void main(String data[])
{
try {
// Get the name of the contact to be updated
// from the Command line argument
String newName = data[ 0 ];
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Checking whether the name of contact exists.
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number
= Long
.parseLong(
nameNumberString
.substring(index + 1 ));
// if condition to find existence of record.
if (name == newName) {
found = true ;
break ;
}
}
// Delete the contact if record exists.
if (found == true ) {
// Creating a temporary file
// with file pointer as tmpFile.
File tmpFile = new File( "temp.txt" );
// Opening this temporary file
// in ReadWrite Mode
RandomAccessFile tmpraf
= new RandomAccessFile(tmpFile, "rw" );
// Set file pointer to start
raf.seek( 0 );
// Traversing the friendsContact.txt file
while (raf.getFilePointer() < raf.length()) {
// Reading the contact from the file
nameNumberString = raf.readLine();
index = nameNumberString.indexOf( '!' );
name = nameNumberString.substring( 0 , index);
// Check if the fetched contact
// is the one to be deleted
if (name.equals(inputName)) {
// Skip inserting this contact
// into the temporary file
continue ;
}
// Add this contact in the temporary file
tmpraf.writeBytes(nameNumberString);
// Add the line separator in the temporary file
tmpraf.writeBytes(System.lineSeparator());
}
// The contact has been deleted now
// So copy the updated content from
// the temporary file to original file.
// Set both files pointers to start
raf.seek( 0 );
tmpraf.seek( 0 );
// Copy the contents from
// the temporary file to original file.
while (tmpraf.getFilePointer() < tmpraf.length()) {
raf.writeBytes(tmpraf.readLine());
raf.writeBytes(System.lineSeparator());
}
// Set the length of the original file
// to that of temporary.
raf.setLength(tmpraf.length());
// Closing the resources.
tmpraf.close();
raf.close();
// Deleting the temporary file
tmpFile.delete();
System.out.println( " Friend deleted. " );
}
// The contact to be deleted
// could not be found
else {
// Closing the resources.
raf.close();
// Print the message
System.out.println( " Input name"
+ " does not exists. " );
}
}
catch (IOException ioe) {
System.out.println(ioe);
}
}
}
输出如下:
编译和删除文件中的联系人:
javac DeleteFriend.java
java DeleteFriend pqr
Friend deleted.
java DeleteFriend tqr
Input name does not exists.
文件:
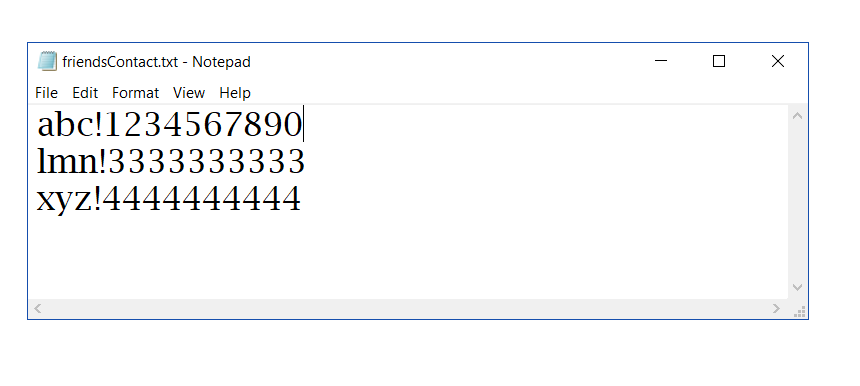
创造
// Java program to create a file "friendsContact.txt"
// and add a new contact in the file
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class AddFriend {
public static void main(String data[])
{
try {
// Get the name of the contact to be updated
// from the Command line argument
String newName = data[ 0 ];
// Get the number to be updated
// from the Command line argument
long newNumber = Long.parseLong(data[ 1 ]);
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Checking whether the name
// of contact already exists.
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number
= Long
.parseLong(
nameNumberString
.substring(index + 1 ));
// if condition to find existence of record.
if (name == newName || number == newNumber) {
found = true ;
break ;
}
}
if (found == false ) {
// Enter the if block when a record
// is not already present in the file.
nameNumberString
= newName
+ "!"
+ String.valueOf(newNumber);
// writeBytes function to write a string
// as a sequence of bytes.
raf.writeBytes(nameNumberString);
// To insert the next record in new line.
raf.writeBytes(System.lineSeparator());
// Print the message
System.out.println( " Friend added. " );
// Closing the resources.
raf.close();
}
// The contact to be updated
// could not be found
else {
// Closing the resources.
raf.close();
// Print the message
System.out.println( " Input name"
+ " does not exists. " );
}
}
catch (IOException ioe) {
System.out.println(ioe);
}
catch (NumberFormatException nef) {
System.out.println(nef);
}
}
}
读
// Java program to read from file "friendsContact.txt"
// and display the contacts
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class DisplayFriends {
public static void main(String data[])
{
try {
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Traversing the file
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number
= Long
.parseLong(
nameNumberString
.substring(index + 1 ));
// Print the contact data
System.out.println( "Friend Name: "
+ name + "\n"
+ "Contact Number: "
+ number + "\n" );
}
catch (IOException ioe)
{
System.out.println(ioe);
}
catch (NumberFormatException nef)
{
System.out.println(nef);
}
}
}
更新
// Java program to update in the file "friendsContact.txt"
// and change the number of an old contact
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class UpdateFriend {
public static void main(String data[])
{
try {
// Get the name of the contact to be updated
// from the Command line argument
String newName = data[ 0 ];
// Get the number to be updated
// from the Command line argument
long newNumber = Long.parseLong(data[ 1 ]);
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Checking whether the name
// of contact already exists.
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number = Long
.parseLong(nameNumberString
.substring(index + 1 ));
// if condition to find existence of record.
if (name == newName || number == newNumber) {
found = true ;
break ;
}
}
// Update the contact if record exists.
if (found == true ) {
// Creating a temporary file
// with file pointer as tmpFile.
File tmpFile = new File( "temp.txt" );
// Opening this temporary file
// in ReadWrite Mode
RandomAccessFile tmpraf
= new RandomAccessFile(tmpFile, "rw" );
// Set file pointer to start
raf.seek( 0 );
// Traversing the friendsContact.txt file
while (raf.getFilePointer() < raf.length()) {
// Reading the contact from the file
nameNumberString = raf.readLine();
index = nameNumberString.indexOf( '!' );
name = nameNumberString.substring( 0 , index);
// Check if the fetched contact
// is the one to be updated
if (name.equals(inputName)) {
// Update the number of this contact
nameNumberString
= name + "!"
+ String.valueOf(newNumber);
}
// Add this contact in the temporary file
tmpraf.writeBytes(nameNumberString);
// Add the line separator in the temporary file
tmpraf.writeBytes(System.lineSeparator());
}
// The contact has been updated now
// So copy the updated content from
// the temporary file to original file.
// Set both files pointers to start
raf.seek( 0 );
tmpraf.seek( 0 );
// Copy the contents from
// the temporary file to original file.
while (tmpraf.getFilePointer() < tmpraf.length()) {
raf.writeBytes(tmpraf.readLine());
raf.writeBytes(System.lineSeparator());
}
// Set the length of the original file
// to that of temporary.
raf.setLength(tmpraf.length());
// Closing the resources.
tmpraf.close();
raf.close();
// Deleting the temporary file
tmpFile.delete();
System.out.println( " Friend updated. " );
}
// The contact to be updated
// could not be found
else {
// Closing the resources.
raf.close();
// Print the message
System.out.println( " Input name"
+ " does not exists. " );
}
}
catch (IOException ioe) {
System.out.println(ioe);
}
catch (NumberFormatException nef) {
System.out.println(nef);
}
}
}
删除
// Java program to delete a contact
// from the file "friendsContact.txt"
import java.io.File;
import java.io.IOException;
import java.io.RandomAccessFile;
import java.lang.NumberFormatException;
class DeleteFriend {
public static void main(String data[])
{
try {
// Get the name of the contact to be updated
// from the Command line argument
String newName = data[ 0 ];
String nameNumberString;
String name;
long number;
int index;
// Using file pointer creating the file.
File file = new File( "friendsContact.txt" );
if (!file.exists()) {
// Create a new file if not exists.
file.createNewFile();
}
// Opening file in reading and write mode.
RandomAccessFile raf
= new RandomAccessFile(file, "rw" );
boolean found = false ;
// Checking whether the name of contact exists.
// getFilePointer() give the current offset
// value from start of the file.
while (raf.getFilePointer() < raf.length()) {
// reading line from the file.
nameNumberString = raf.readLine();
// finding the position of '!'
index = nameNumberString.indexOf( '!' );
// separating name and number.
name = nameNumberString
.substring( 0 , index);
number
= Long
.parseLong(
nameNumberString
.substring(index + 1 ));
// if condition to find existence of record.
if (name == newName) {
found = true ;
break ;
}
}
// Delete the contact if record exists.
if (found == true ) {
// Creating a temporary file
// with file pointer as tmpFile.
File tmpFile = new File( "temp.txt" );
// Opening this temporary file
// in ReadWrite Mode
RandomAccessFile tmpraf
= new RandomAccessFile(tmpFile, "rw" );
// Set file pointer to start
raf.seek( 0 );
// Traversing the friendsContact.txt file
while (raf.getFilePointer() < raf.length()) {
// Reading the contact from the file
nameNumberString = raf.readLine();
index = nameNumberString.indexOf( '!' );
name = nameNumberString.substring( 0 , index);
// Check if the fetched contact
// is the one to be deleted
if (name.equals(inputName)) {
// Skip inserting this contact
// into the temporary file
continue ;
}
// Add this contact in the temporary file
tmpraf.writeBytes(nameNumberString);
// Add the line separator in the temporary file
tmpraf.writeBytes(System.lineSeparator());
}
// The contact has been deleted now
// So copy the updated content from
// the temporary file to original file.
// Set both files pointers to start
raf.seek( 0 );
tmpraf.seek( 0 );
// Copy the contents from
// the temporary file to original file.
while (tmpraf.getFilePointer() < tmpraf.length()) {
raf.writeBytes(tmpraf.readLine());
raf.writeBytes(System.lineSeparator());
}
// Set the length of the original file
// to that of temporary.
raf.setLength(tmpraf.length());
// Closing the resources.
tmpraf.close();
raf.close();
// Deleting the temporary file
tmpFile.delete();
System.out.println( " Friend deleted. " );
}
// The contact to be deleted
// could not be found
else {
// Closing the resources.
raf.close();
// Print the message
System.out.println( " Input name"
+ " does not exists. " );
}
}
catch (IOException ioe) {
System.out.println(ioe);
}
}
}